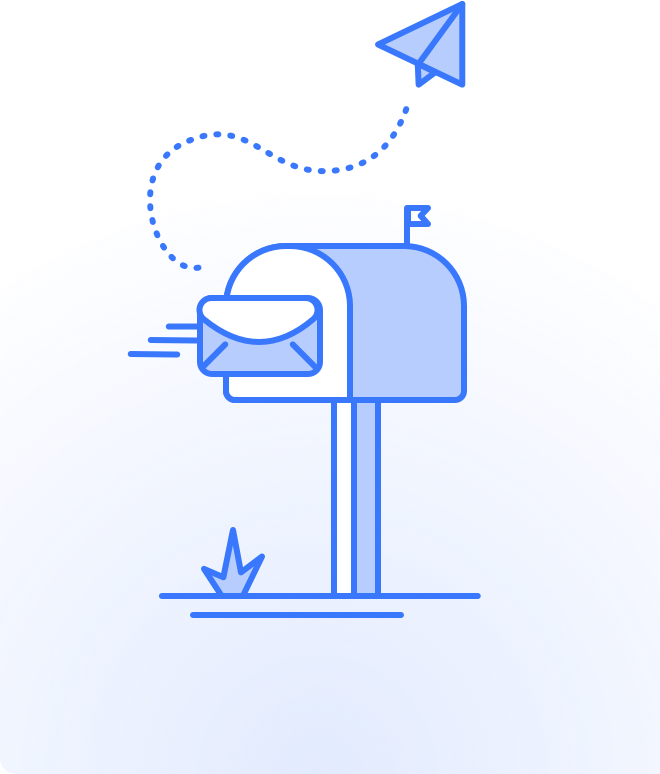
Imagine the ability to enhance your app’s communication by seamlessly adapting the tone and style of your messages with a single click. With QuickBlox’s new Android library – AI Rephrase– this innovative capability is within your reach. This is an incredibly simple, user-friendly, and lightweight library that works out of the box and encompasses all the essential functionalities for transforming the tone of a message whether you’re aiming for a formal or friendly vibe, or any other style.
We want to make AI-powered chat in Android apps accessible for developers, that’s why we’ve made is easy to integrate our AI library into any of your Android applications with a few simple clicks. You also have the option to create Android messaging apps with AI Rephrase by using the QuickBlox Android UI Kit.
To use AI Rephrase, you’ll need to select the tone with which you’d like to rephrase the text and pass both the chosen tone and the text itself to the library.
The overall workflow of the library is depicted in the diagram below:
Let’s examine the stages of operation in more detail:
Stage 1 – Any Android application which uses the QuickBlox AI Rephrase library. The application can communicate with and use the library API.
Stage 2 – The QuickBlox AI Rephrase library which contains predefined tones and has logic for adding and removing tones. A reset mechanism is also available when a user wants to reset all tones to the default state.
The library can communicate with OpenAI through a QuickBlox Proxy Server or by a direct request.
Stage 3A – QuickBlox Proxy Server which stores the OpenAI Token and works via a QuickBlox Token. We strongly recommended this flow because it offers better security and you don’t need to save the OpenAI API Token inside the application.
Stage 3B – Direct request method means your data goes directly to OpenAI API without any proxy. We strongly recommend that you only use this method for development or debugging, because this variant requires you to store the OpenAI Api key within your application.
Stage 4 – OpenAI API, provides users with access to pre-trained AI models.
Android AI Rephrase Library
QuickBlox AI Documentation
Implementing this feature is easy. To utilize the QuickBlox AI Rephrase library, you only need to follow a few simple steps.
You first need to add the repository to your existing Android application.
Open Gradle (root level) file and add the code as illustrated in the code snippet below. See the dependencyResolutionManagement section in particular:
dependencyResolutionManagement { repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS) repositories { google() mavenCentral() // below we have the a link to QuickBlox AI repository maven { url= "https://github.com/QuickBlox/public-ai-releases" } } }
Next, you need to add a dependency to the existing Android application.
Open Gradle (application level) file and add the code as illustrated in the snippet below. See the dependencies section.
dependencies { // some other dependencies // below we have a link to QuickBlox AI Rephrase library Implementation "com.quickblox:android-ai-editing-assistant:2.1.0" }
Version 2.1.0 can be updated to the latest version. Discover the latest release and the review the entire release history in our repository: https://github.com/QuickBlox/android-ai-releases/releases.
The QuickBlox AI Rephrase library can be used with two different approaches. Either directly, using the library with raw Open AI token, or with a QuickBlox session token via a proxy.
Direct approach – While this method may be suitable during the development phase, (because it allows the quick testing of functionality without having to spend time rolling out the proxy server), it’s not recommended when the app is released. This is because care should always be taken to hide tokens, for example, by adding the token to the local.properties file. But this direct approach involves using an OpenAI token directly by allowing our the AI library to directly communicate with the OpenAI API.
Proxy approach – This is the preferred method. It is far more secure because the OpenAI token is encapsulated inside the proxy server. Using a QuickBlox session token, the AI library will communicate via a proxy server (not directly) with OpenAI API.
To invoke the QuickBlox AI Rephrase library method you need to add necessary imports and invoke the executeByOpenAIToken method like in the code snippet below.
// below we have required imports for QuickBlox AI Rephrase library import com.quickblox.android_ai_editing_assistant.QBAIRephrase import com.quickblox.android_ai_editing_assistant.callback.Callback import com.quickblox.android_ai_editing_assistant.exception.QBAIRephraseException import com.quickblox.android_ai_editing_assistant.model.ToneImpl import com.quickblox.android_ai_editing_assistant.settings.RephraseSettingsImpl import com.quickblox.android_ai_editing_assistant.settings.RephraseSettingsImpl.Model /* * some code */ private fun rephraseWithApiKey() { val toneName = "Professional" val toneDescription = "tone description" val tone = ToneImpl(toneName, toneDescription) val openAIToken = "open_ai_token" // our raw open ai token val rephraseSettings = RephraseSettingsImpl(openAIToken, tone) // optional parameters val maxRequestTokens = 3000 // default value val openAIModel = Model.GPT_3_5_TURBO // default value val temperature = 0.5f // default value rephraseSettings.setMaxRequestTokens(maxRequestTokens) rephraseSettings.setModel(openAIModel) rephraseSettings.setTemperature(temperature) val text = "Hi Bro. Can you help me?" QBAIRephrase.rephraseAsync(text, rephraseSettings, object : Callback { override fun onComplete(result: String) { // here is be result as the answer } override fun onError(error: QBAIRephraseException) { // oops, something goes wrong } }) }
// below we have required imports for QuickBlox AI Rephrase library import com.quickblox.android_ai_editing_assistant.QBAIRephrase import com.quickblox.android_ai_editing_assistant.callback.Callback import com.quickblox.android_ai_editing_assistant.exception.QBAIRephraseException import com.quickblox.android_ai_editing_assistant.model.ToneImpl import com.quickblox.android_ai_editing_assistant.settings.RephraseSettingsImpl import com.quickblox.android_ai_editing_assistant.settings.RephraseSettingsImpl.Model /* * some code */ private fun rephraseWithProxyServer() { val toneName = "Professional" val toneDescription = "tone description" val tone = ToneImpl(toneName, toneDescription) val qbToken = "quickblox_token" // our QuickBlox session token val serverProxyUrl = "https://my.proxy-server.com" // our proxy server url val rephraseSettings = RephraseSettingsImpl(qbToken, serverProxyUrl, tone) // optional parameters val maxRequestTokens = 3000 // default value val openAIModel = Model.GPT_3_5_TURBO // default value val temperature = 0.5f // default value rephraseSettings.setMaxRequestTokens(maxRequestTokens) rephraseSettings.setModel(openAIModel) rephraseSettings.setTemperature(temperature) val text = "Hi Bro. Can you help me?" QBAIRephrase.rephraseAsync(text, rephraseSettings, object : Callback { override fun onComplete(result: String) { // here is be result as the answer } override fun onError(error: QBAIRephraseException) { // oops, something goes wrong } }) }
We recommended using the proxy server method. Please ensure you use the latest method which you can in our repository: https://github.com/QuickBlox/qb-ai-assistant-proxy-server/releases
To set up the rephrase tone, you need to set this Tone in the constructor of the object RephraseSettingsImpl.
val toneName = "Professional" val toneDescription = "tone description" val tone = ToneImpl(toneName, toneDescription) val openAIToken = "open_ai_token" // our raw open ai token val rephraseSettings = RephraseSettingsImpl(openAIToken, tone)
Exception: In the QuickBlox AI Rephrase library you could receive QBAIRephraseException in onError callback. You can get detailed information from the message field, as shown in the code snippet below:
override fun onError(error: QBAIRephraseException) { // oops, something goes wrong }
The QuickBlox development team has integrated the AI Rephrase library into the QuickBlox Android UI Kit to save you time and make it easier to use the library.
To learn how to implement the QuickBlox Android UI Kit and send your first message, read our official documentation.
Also be sure that you have a QuickBlox account.
Include references to the SDK repositories in your project-level build.gradle file at the root directory or to the settings.gradle file in your Android application.
Specify the URL of the QuickBlox repository where the files are stored. By following this URL, Gradle can find the SDK artifacts:
repositories { // below we have the link to QuickBlox UIKit repository maven { url "https://github.com/QuickBlox/android-ui-kit-releases/raw/master/" } // below we have the link to QuickBlox Android SDK repository maven { url "https://github.com/QuickBlox/quickblox-android-sdk-releases/raw/master/" } // below we have the link to QuickBlox AI repository maven { url "https://github.com/QuickBlox/android-ai-releases/raw/main/" } }
Next you need to add the implementation of QuickBlox dependencies in your module-level(App) build.gradle file in your Android application.
dependencies { implementation "com.quickblox:android-ui-kit:0.7.0" }
By default, AI Rephrase is turned on in the UI Kit, but not configured.
To configure AI Rephrase using the OpenAI Token, you need to invoke the following method in the UI Kit entry point after invoking the init() method.
val openAIToken = "open_ai_token" QuickBloxUiKit.init(applicationContext) QuickBloxUiKit.enableAIRephraseWithOpenAIToken(openAIToken)
To configure AI Rephrase with the Proxy server you need to invoke the following method in the UI Kit entry point after you invoke the init() method.
val serverProxyUrl = "https://my.proxy-server.com" QuickBloxUiKit.init(applicationContext) QuickBloxUiKit.enableAIRephraseWithProxyServer(serverProxyUrl)
To disable AI Rephrase you need to invoke the method in the UI Kit entry point after we invoke the init() method.
QuickBloxUiKit.init(applicationContext) // below we disable the AI Rephrase QuickBloxUiKit.disableAIRephrase()
To make it super easy to use AI Rephrase within as part of the QuickBlox Android UI Kit, we have prepared several Rephrase use cases.
You can choose either the direct operations, or operations through a proxy approach when using the AI Rephrase Library. Both approaches are accepted in the constructor AIRephraseToneEntity, to rephrase outgoing text.
For asynchrony in the Android UI Kit, we use a Kotlin Coroutine. To this end, we use the viewModelScope to launch a new coroutine using the launch() builder.
A ViewModelScope is defined for each ViewModel in your app. Any coroutine launched in this scope is automatically canceled if the ViewModel is cleared.
Learn more about, How to use Kotlin Coroutines in Android
viewModelScope.launch { try { val toneName = "Professional" val description = "Tone description" val tone = AIRephraseToneEntityImpl(toneName, description) val entity = AIRephraseEntityImpl(tone) val text = "Hi Bro. Can you help me?" entity.setOriginalText(text) val resultEntity = LoadAIRephraseWithApiKeyUseCase(dialogId, entity).execute() val rephrasedText = resultEntity?.getRephrasedText() } catch (exception: DomainException) { // error handling } }
viewModelScope.launch { try { val toneName = "Professional" val description = "Tone description" val tone = AIRephraseToneEntityImpl(toneName, description) val entity = AIRephraseEntityImpl(tone) val text = "Hi Bro. Can you help me?" entity.setOriginalText(text) val resultEntity = LoadAIRephraseWithProxyServerUseCase(dialogId, entity).execute() val rephrasedText = resultEntity?.getRephrasedText() } catch (exception: DomainException) { // error handling } }
The QuickBlox UIKit for Android allows users to rephrase chat messages into different tones in a matter of seconds. The QuickBlox UIKit already features 10 preconfigured text formatting options, which include:
Moreover, if the provided preset options are insufficient, you can effortlessly add your own. This process is also quite straightforward. Of course, you are also able to delete any of the options as desired.
In QuickBlox UIKit we have AIRephraseToneEntity interface.
The AIRephraseToneEntity interface has three methods:
All methods return String. The QuickBlox UIKit already has implementation of interface with class name AIRephraseToneEntityImpl which you can use to build custom tone. The class have all parameters in constructor. They are: name (required), description (required), icon (optional).
As we can see the class has only two required parameters name and description.
There are 2 available methods to work with tones in QuickBloxUiKit entry point.
getRephraseTones() – return list of AIRephraseToneEntity interface
Code snippet:
private fun getTones() { val tones: List= QuickBloxUiKit.getRephraseTones() // tones - it's list of implementation AIRephraseToneEntity interfaces }
setRephraseTones(rephraseTones) – This method allows you to specify a list of tones of your own. It is also possible to modify an already existing list and set it using this method.
Code snippet:
private fun setTones() { val tones = mutableListOf() tones.add(AIRephraseToneEntityImpl("Professional", "Professional tone description", "\uD83D\uDC54")) tones.add(AIRephraseToneEntityImpl("Friendly", "Friendly tone description", "\uD83E\uDD1D")) QuickBloxUiKit.setRephraseTones(tones) }
That’s it! You’re all set.
This tutorial aims to enhance your Android chat application with the powerful AI Rephrase library. Whether you’ve chosen to integrate it as a standalone library, seamlessly weave it into your existing app, or leverage its capabilities through the Android UI Kit from QuickBlox, you’re now equipped to revolutionize the way users communicate within your app.
QuickBlox is committed to building new tools to aid developers with AI chat integration for Android apps. In addition to AI Rephrase, you can also explore AI Answer Assist and AI Translate, two further AI enhancements for Android chat applications:
AI Translate Implementation Guide for Android Apps
AI Answer Assist Implementation Guide for Android Apps
Interested in chatbot integration for Android? Read about our soon-to-be released SmartChat Assistants. This Android chatbot solution allows you to easily build and add OpenAI-integrated chatbots to any Android app.